Here is the Python program:
import re # module for regular expressions
search_string='''This is a string to search for a regular expression like regular expression or regular-expression or regular:expression or regular&expression''' #string to search for a regular expression
pattern = "regular.expression" #Assigns the regular expression to pattern
substitution="regular expression" #substitute all occurrences of pattern with regular expression string stored in substitution
replace_results = re.sub(pattern,substitution,search_string) # sub() method from the re package to substitute all occurrences of the pattern with substitution
print(replace_results) #Assigns the outcome of the sub() method to this variable
Explanation:
This is a string to search for a regular expression like regular expression or regular-expression or regular:expression or regular&expression
search_string='''This is a string to search for a regular expression like regular expression or regular-expression or regular:expression or regular&expression'''
The following statement assigns the regular expression to a variable named pattern
.
pattern = "regular.expression"
The following statement is used to substitute the pattern (regular expression) in the search_string by replacing all occurrences of "regular expression" sub-string on search_string.
substitution="regular expression"
The following statement uses re.sub() method to replace all the occurrences of a pattern with another sub string ("regular expression"). This means in search_string, the sub strings like regular expression, regular-expression, regular:expression or regular&expression are replaced with string "regular expression". This result is stored in replace_results variable. Three arguments are passed to re.sub() method:
sub string to replace i.e. pattern
sub string to replace with i.e. substitution
The actual string i.e. search_string
replace_results = re.sub(pattern,substitution,search_string)
The following print statement displays the output of replace_results
print(replace_results)
The output of the above program is:
This is a string to search for a regular expression like regular expression or regular expression or regular expression or regular expression
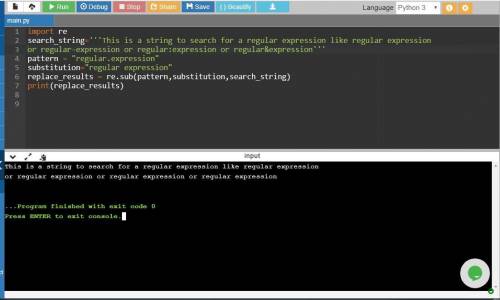